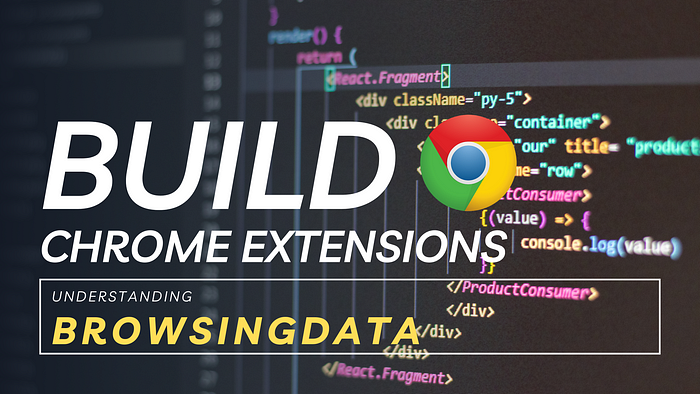
The chrome.browsingData
API deals with removing browsing data from the local user’s browser. This includes caches, cookies, downloads, passwords, history and many more. You can see a comprehensive list of browser data the chrome extension can remove using this API here: https://developer.chrome.com/docs/extensions/reference/api/browsingData#method-remove
How to declare it in Manifest.json
{
...
"permissions": [
"browsingData",
],
...
}
How to clear Browsing Data
You’ll write the code in your background scripts or extension pages (popup’s JavaScript) as the chrome.browsingData
API does NOT working it content scripts.
There are many remove functions you can call, each of a specify type of browsing data. We cannot cover them all. Rather it would be more effective to explain the parameters you put in each of those functions.
Let’s clear the cookies for example.
This code snippet will remove cookies only from Google since Fri Aug 30 2024.
chrome.browsingData.removeCookies({
origins:["https://google.com"],
// excludeOrigins:["https://google.com"], // cannot mix it with origins
since: 1725024698180, // optional epoch number
}, () => {
console.log("Clearing Cookies Done");
})
Let’s clear downloads
This code snippet will remove downloads (NOT the files) only from Google since Fri Aug 30 2024.
chrome.browsingData.removeDownloads({
origins:["https://google.com"],
// excludeOrigins:["https://google.com"], // cannot mix it with origins
since: 1725024698180, // optional epoch number
}, () => {
console.log("Clearing Cookies Done");
})
How about remove multiple different type browsing data
For that we use the chrome.broswerData.remove
function.
const options = {
origins: ["https://google.com"],
// excludeOrigins:["https://google.com"], // cannot mix it with origins
since: 1725024698180, // optional epoch number
};
// what kind of browser data
const dataToRemove = {
appcache: true,
cache: true,
cacheStorage: true,
cookies: false,
downloads: false,
fileSystems: true,
formData: true,
history: false,
indexedDB: true,
localStorage: true,
passwords: false,
serverBoundCertificates: true,
serviceWorkers: false,
webSQL: true,
};
//remove browsering data
chrome.browsingData.remove(options, dataToRemove, () => {
console.log("Browsing data cleared");
});
Notice that each of the browsingData options has its own dedicated function. For instance,
cookies
haschrome.broswingData.removeCookies
,history
haschrome.broswingData.removeHistory
Sample Project
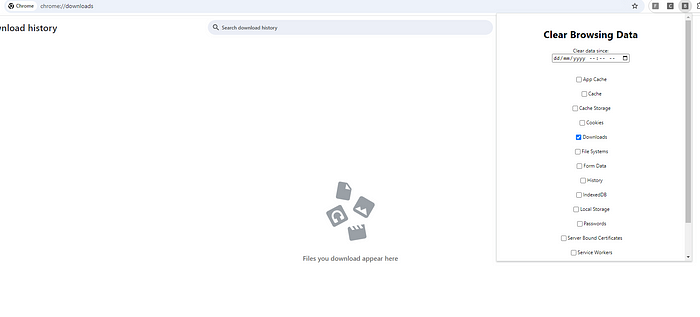
We are going to create a chrome extension that a user will select the browsing data the want to clear and the date-time to start the removal. Here is the source code for the project: https://github.com/BuildChromeExtensions/browserdatacleaner
We are going to need 3 files for this one. A manifest.json
, popup.html
and popup.js
.
manifest.json
Note: Do not forget to set the
host_permissions
for the websites you might be clear browsing data from.
{
"name": "Browsing Data Cleaner",
"version": "1.0.0.0",
"manifest_version": 3,
"action": {
"default_popup": "popup.html"
},
"host_permissions": [
"<all_urls>"
],
"permissions": [
"browsingData"
]
}
popup.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style type="text/css">
body {
width: 400px;
height: 530px;
padding: 10px 20px;
}
form {
display: flex;
flex-direction: column;
align-items: center;
}
label {
display: flex;
}
button {
background: #03c2fc;
color: #ffffff;
padding: 10px 20px;
border: none;
cursor: pointer;
box-shadow: rgba(100, 100, 111, 0.2) 0px 7px 29px 0px;
transition: all 0.4s;
border-radius: 30px;
font-weight: 700;
}
button:hover {
background: #026482;
}
</style>
</head>
<body>
<form id="clear-data-form">
<h1>Clear Browsing Data</h1>
<label for="date">Clear data since:</label>
<input type="datetime-local" id="date" name="date" required><br><br>
<label><input type="checkbox" name="appcache"> App Cache</label><br>
<label><input type="checkbox" name="cache"> Cache</label><br>
<label><input type="checkbox" name="cacheStorage"> Cache Storage</label><br>
<label><input type="checkbox" name="cookies"> Cookies</label><br>
<label><input type="checkbox" name="downloads"> Downloads</label><br>
<label><input type="checkbox" name="fileSystems"> File Systems</label><br>
<label><input type="checkbox" name="formData"> Form Data</label><br>
<label><input type="checkbox" name="history"> History</label><br>
<label><input type="checkbox" name="indexedDB"> IndexedDB</label><br>
<label><input type="checkbox" name="localStorage"> Local Storage</label><br>
<label><input type="checkbox" name="passwords"> Passwords</label><br>
<label><input type="checkbox" name="serverBoundCertificates"> Server Bound Certificates</label><br>
<label><input type="checkbox" name="serviceWorkers"> Service Workers</label><br>
<label><input type="checkbox" name="webSQL"> WebSQL</label><br><br>
<button type="submit">Clear Data</button>
</form>
<script type="text/javascript" src="./popup.js"></script>
</body>
</html>
popup.js
// https://developer.chrome.com/docs/extensions/reference/api/browsingData
document.querySelector('form').onsubmit = function (e) {
e.preventDefault();
const date = e.target.date.value;
const since = new Date(date).getTime();
const appcache = e.target.appcache.checked;
const cache = e.target.cache.checked;
const cacheStorage = e.target.cacheStorage.checked;
const cookies = e.target.cookies.checked;
const downloads = e.target.downloads.checked;
const fileSystems = e.target.fileSystems.checked;
const formData = e.target.formData.checked;
const history = e.target.history.checked;
const indexedDB = e.target.indexedDB.checked;
const localStorage = e.target.localStorage.checked;
const passwords = e.target.passwords.checked;
const serverBoundCertificates = e.target.serverBoundCertificates.checked;
const serviceWorkers = e.target.serviceWorkers.checked;
const webSQL = e.target.webSQL.checked;
const options = {
since: since,
};
// what kind of browser data
const dataToRemove = {
appcache: appcache,
cache: cache,
cacheStorage: cacheStorage,
cookies: cookies,
downloads: downloads,
fileSystems: fileSystems,
formData: formData,
history: history,
indexedDB: indexedDB,
localStorage: localStorage,
passwords: passwords,
serverBoundCertificates: serverBoundCertificates,
serviceWorkers: serviceWorkers,
webSQL: webSQL,
};
//remove browsering data
chrome.browsingData.remove(options, dataToRemove, () => {
alert("Browsing data cleared");
});
}