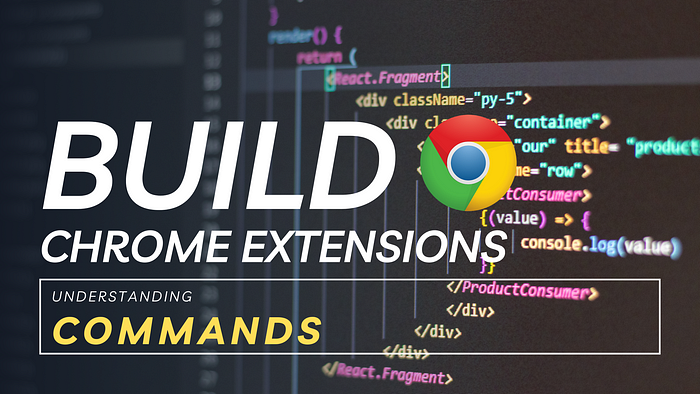
The chrome.commands
API is used to enable the chrome extension run actions from keyboard shortcuts. You can bind a defined-command with a combinations for keyboard keys. Let’s show you had it is done.
You find the official documentation by the Google Chrome Team here: https://developer.chrome.com/docs/extensions/reference/api/commands
How to Declare it in Manifest.json
You’ll notice the commands
keyword is not in permissions
array.
{
"name": "My extension",
"permissions":[],
...
"commands": {
"run_my_code": {
"suggested_key": {
"default": "Ctrl+Shift+Y",
"mac": "Command+Shift+Y"
},
"description": "Run Your code on the current page."
}
},
...
}
In this code snippet we have a command call run_my_code
and we’ve specified the suggested_key
as Ctrl+Shift+Y
. You can specify what suggested_key
should be for each platform; windows
, mac
, chromeos
and linux
.
Yes, for those of you who are asking, you can have more than one command defined. You just need to add another object with the same structure in the commands
object. See run_my_other_code
in the code snippet below.
{
...
"commands": {
"run_my_code": {
"suggested_key": {
"default": "Ctrl+Shift+Y",
"mac": "Command+Shift+Y"
},
"description": "Run Your code on the current page."
},
"run_my_other_code": {
"suggested_key": {
"windows": "Ctrl+Shift+Y",
"mac": "Command+Shift+Y",
"chromeos": "Ctrl+Shift+U",
"linux": "Ctrl+Shift+J"
},
"description": "Run My other code on the current page."
}
},
...
}
What Suggested Keys Are Supported?
Not all keys can be used as suggested_key
. Here are the following keys that supported.
- Letters:
A-Z
, - Numbers:
0–9
- Standard Keys:
Comma
,Period
,Home
,End
,PageUp
,PageDown
,Space
,Insert
,Delete
,Up
,Down
,Left
,Right
,MediaNextTrack
,MediaPlayPause
,MediaPrevTrack
,MediaStop
- Modifier Keys:
Ctrl
,Alt
,Shift
for macOSOption
,MacCtrl
,Command
Rules for Key combinations
- Commands must have one
Ctrl
orAlt
modifier key. - Mixing them with
Shift
is optional but do not start withShift
. - Finish the combinations with one Number Key, Letter Key or Standard Key
- For
mac
use the macOS modifiers keys.
Good Examples
{
...
"commands": {
"run_my_other_code": {
"suggested_key": {
"windows": "Ctrl+Shift+Y",
"mac": "Command+Shift+Y",
"chromeos": "Ctrl+Home",
"linux": "Alt+MediaNextTrack"
},
"description": "Run My other code on the current page."
}
},
...
}
Bad Examples
{
...
"commands": {
"run_my_other_code": {
"suggested_key": {
"windows": "L+1",
"mac": "Ctrl+Shift+Y",
"chromeos": "Ctrl+Alt",
"linux": "Shift+T"
},
"description": "Run My other code on the current page."
}
},
...
}
Action Command
As you can see about we are free to name the commands; run_my_code
run_my_other_code
. However there is a special command dedication for extension’s action icon/popup on the toolbar; _execute_browser_action
. Essential you can open the extensions with a shortcut key.
{
...
"commands": {
"_execute_action": {
"suggested_key": {
"default": "Ctrl+U"
},
"description": "Open the extension"
}
},
...
}
or
{
...
"commands": {
"_execute_action": {
"suggested_key": "Ctrl+U",
"description": "Open the extension"
}
},
...
}
What if the shortcuts already defined in another extensions?
If two extensions have the same shortcut keys defined. The first extension installed will be the one that works.
How to know which commands are take?
You can run this code at install time to see which commands have a shortcut. A snippet from the Google Chrome Team https://developer.chrome.com/docs/extensions/reference/api/commands#verify_commands_registered
chrome.runtime.onInstalled.addListener((details) => {
if (details.reason === chrome.runtime.OnInstalledReason.INSTALL) {
checkCommandShortcuts();
}
});
// Only use this function during the initial install phase. After
// installation the user may have intentionally unassigned commands.
function checkCommandShortcuts() {
chrome.commands.getAll((commands) => {
let missingShortcuts = [];
for (let {name, shortcut} of commands) {
if (shortcut === '') {
missingShortcuts.push(name);
}
}
if (missingShortcuts.length > 0) {
// Update the extension UI to inform the user that one or more
// commands are currently unassigned.
}
});
}
What if you want to use those shortcuts anyway?
If you are stubborn like we are and want use a certain shortcuts combination but it is already registered by another extension, there are 2 solutions.
First is uninstalling that other extension, but this hasn’t been a popular solution.
Second is by add a keypress listener in your content scripts. It is similar code you would write in your website code to handle key events, only that you are running it in the content scripts. This way, registered in the other extension or not your key presses trigger.
document.body.onkeydown = (e) => {
if (e.altKey && e.key === 'l') {
// ALT+L
} else if (e.altKey && e.key === 'q') {
// ALT+Q
}
}
However there is an obvious limitation to this approach. A webpage has to be opened for this to work.
You can see the commands defined by your extensions if you go this link in your chrome browser
chrome://extensions/shortcuts
Handling Commands in Code
// In your background script / service worker
chrome.commands.onCommand.addListener((command, tab) => {
if(command == "run_my_code"){
// run your code.
}
});
Sample Project
We are going to make a chrome extension that opens the popup when a user enters Alt+U
and Facebook Tab when the enter Alt+Shift+F
. Will need 3 files for this one. index.html
, manifest.json
and background.js
the popup UI manifest files and service work (background script) respectively.
You can get access to the source code for the project here: https://github.com/BuildChromeExtensions/chromeCommands
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
width: 200px;
height: 300px;
display: flex;
justify-content: center;
background: purple;
color: white;
text-align: center;
}
</style>
</head>
<body>
<h2>Popup Opened by Shortcut</h2>
</body>
</html>
manifest.json
{
"name": "My Shortcuts",
"manifest_version": 3,
"version": "1.0.0.0",
"permissions": [
"tabs"
],
"action": {
"default_popup": "index.html"
},
"commands": {
"_execute_action": {
"suggested_key": "Alt+U",
"description": "Open the extension"
},
"open_facebook": {
"suggested_key": "Alt+Shift+F",
"description": "Open Facebook"
}
},
"background": {
"service_worker": "background.js"
}
}
background.js
// when shortcut keys pressed
chrome.commands.onCommand.addListener((command, tab) => {
if (command == "open_facebook") {
// open facebook tab
chrome.tabs.create({ url: "https://facebook.com" })
}
});