
The chrome.action
API is used to control everything to do with the your extensions icon found in the toolbar. It encompasses the title, icon, when it is clicked and much more. You can read the Chrome.API documentation on actions more details here https://developer.chrome.com/docs/extensions/reference/api/action

In pervious article we wrote about Understanding Chrome Extensions Permissions we briefly touched on how to declared it in the manifest.json
file. In this article, take a deep dive into the different options available to you. Below is a sample declaration for the action permission.
{
"name": "Chrome Action",
...
"action": {
"default_icon": {
"16": "images/icon16.png",
"24": "images/icon24.png",
"32": "images/icon32.png"
},
"default_title": "Chrome Action Click Me",
"default_popup": "popup.html"
},
...
}
Keep in mind that the action
key is in the manifest.json
is NOT mandatory. So when it is not include the extension action icon defaults to a generic icon.

Options of Action
Lets talk about the different options of the action.
default_icon
is self explanatory, as it specifies what icon to use at what resolutions. Depending on the monitor’s resolution the default_icon
should up differently. Note that the path to the image file should be relative to the location for the manifest.json
As general rule please use
.png
images, do NOT use.gif
,.ico
,.bmp
default_icon
should not confused with icons
{
"name": "Chrome Action",
...
"action": {
"default_icon": {
"16": "images/icon16.png",
"24": "images/icon24.png",
"32": "images/icon32.png"
},
"default_title": "Chrome Action Click Me",
"default_popup": "popup.html"
},
"icons": {
"16": "icon16.png",
"32": "icon32.png",
"48": "icon48.png",
"128": "icon128.png"
}
...
}
icons
is used to to display the icon that shows up in the chrome extensions page in settings. chrome://extensions/
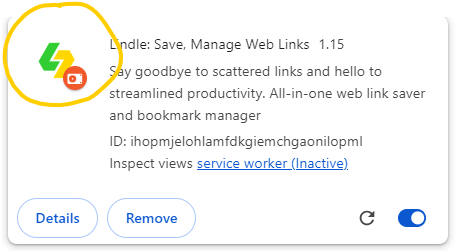
default_title
on the hand, default with the tooltip text that appears when you hover your mouse on the action icon.

default_popup
points the the HTML file that should open when the action icon is clicked.

Sample Extension
You can clone the example the Google Team provides. https://github.com/GoogleChrome/chrome-extensions-samples
The Chrome extension will we be building, changes the action icon, badge text and popup ui randomly when clicked. When the extension is opened the popup will have a button that does the same thing. You can clone the project we are about to show you here. https://github.com/BuildChromeExtensions/chromeaction
We are going to use several different functions from chrome.action to change details about the icon. For our project ensure that you have this file structure.

manifest.json
Notice that we have not put a default_popup
{
"name": "Chrome Action",
"manifest_version": 3,
"version": "1.0.0.0",
"action": {
"default_icon": {
"16": "./icons/icon.png",
"24": "./icons/icon.png",
"32": "./icons/icon.png"
},
"default_title": "Chrome Action Click Me"
},
"background": {
"service_worker": "background.js"
}
}
popup1.html
and popup2.html
Popup1
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chrome Action</title>
<style>
body {
width: 200px;
height: 200px;
padding: 20px;
margin: 0 auto;
display: flex;
justify-content: center;
flex-direction: column;
background-color: goldenrod;
}
</style>
</head>
<body>
<button>Popup 1</button>
<script src="../scripts/extension.js"></script>
</body>
</html>
Popup2
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chrome Action</title>
<style>
body {
width: 300px;
height: 300px;
padding: 20px;
margin: 0 auto;
display: flex;
justify-content: center;
flex-direction: column;
background-color: #4a0576;
}
</style>
</head>
<body>
<button>Popup 2</button>
<script src="../scripts/extension.js"></script>
</body>
</html>
background.js
chrome.action.onClicked.addListener((tab) => {
// names
const names = ['Charles', 'John', 'Pearl', 'Aku', 'Phiri', 'Zak'];
const colors = ['#4A90E2', '#F5A623', '#7ED321', '#9013FE', '#50E3C2', '#B8E986', '#F8E71C', '#D0021B'];
// random index
const indexName = parseInt(Math.random() * names.length)
const indexColor = parseInt(Math.random() * colors.length)
const popupIndex = (1 + parseInt(Math.random() * 2)).toString();
// select name
const name = names[indexName];
// select random number between 0 and 100
const number = parseInt(Math.random() * 100).toString();
// select color
const color = colors[indexColor];
// icon path
const iconPath = chrome.runtime.getURL(`icons/icon${popupIndex}.png`);
// change action properties
chrome.action.setTitle({ title: name });
chrome.action.setIcon({ path: iconPath });
chrome.action.setPopup({ popup: `ui/popup${popupIndex}.html` });
chrome.action.setBadgeText({ text: number });
chrome.action.setBadgeTextColor({ color: "#ffffff" });
chrome.action.setBadgeBackgroundColor({ color: color });
// more change done just look at - https://developer.chrome.com/docs/extensions/reference/api/action
});
extension.js
document.querySelector('button').onclick = (e) => {
// names
const names = ['Charles', 'John', 'Pearl', 'Aku', 'Phiri', 'Zak'];
const colors = ['#4A90E2', '#F5A623', '#7ED321', '#9013FE', '#50E3C2', '#B8E986', '#F8E71C', '#D0021B'];
// random index
const indexName = parseInt(Math.random() * names.length)
const indexColor = parseInt(Math.random() * colors.length)
const popupIndex = (1 + parseInt(Math.random() * 2)).toString();
// select name
const name = names[indexName];
// select random number between 0 and 100
const number = parseInt(Math.random() * 100).toString();
// select color
const color = colors[indexColor];
// icon path
const iconPath = chrome.runtime.getURL(`icons/icon${popupIndex}.png`);
// change action properties
chrome.action.setTitle({ title: name });
chrome.action.setIcon({ path: iconPath });
// remove popup UI on that the chrome.action.onClick can work in the background.js
// that function does not work if the popup ui exists
chrome.action.setPopup({ popup: "" });
chrome.action.setBadgeText({ text: number });
chrome.action.setBadgeTextColor({ color: "#ffffff" });
chrome.action.setBadgeBackgroundColor({ color: color });
// more change done just look at - https://developer.chrome.com/docs/extensions/reference/api/action
}

You can do even more interesting things like open the popup in a particular window and tab using the chrome.action.openPopup
function. See https://developer.chrome.com/docs/extensions/reference/api/action#method-openPopup.
Note: if there is a default_popup set the chrome.action.onClick does not work, because it overrides the onClick function open the popup. But if there is no popup set then chrome.action.onClick should work. chrome.action function are one of many that only work in popup javascript files and background scripts NOT in content scripts.
Leave a Reply