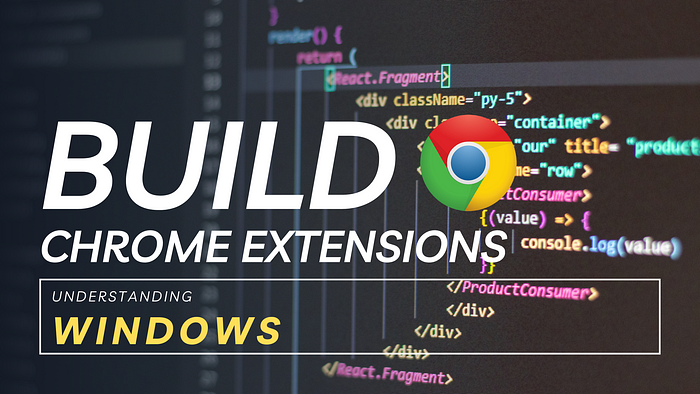
In this article we are going to look at the chrome.windows
API. Its an API used for interacting with the browser windows. Similar to the chrome.tabs
API you can use the API to create and change browser windows.
Types of Windows
There are different types of windows you can create.
Popup or Panel
These are borderless windows. You can not add tabs to these kind of windows. According to the documentation โpanel
is deprecated and is available only to existing allowlisted extensions on Chrome OS.โ https://developer.chrome.com/docs/extensions/reference/api/windows#type-WindowState
So youโll use popup
for your window type and not panel
.
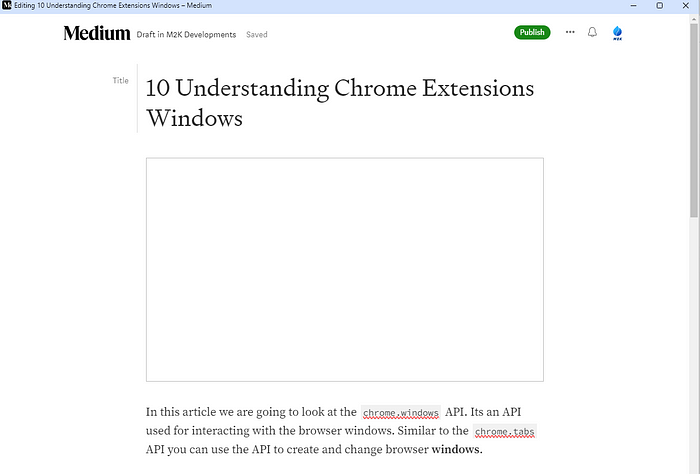
Normal
These are the regular windows with the URL bar at the top.
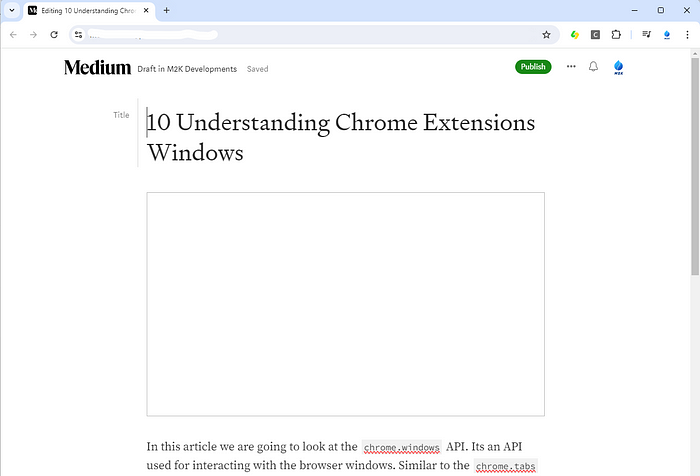
How to declare in manifest.json
Technically you do not need to declare anything in the manifest.json
to use the chrome.windows
API. The tabs
permission is NOT mandatory. We add because windows consists of tabs and if you want to also include tab information when getting the window objects the tabs
permission needs to be set.
{
"name": "Chrome Windows",
"version": "1.0.0.0",
"description": "A chrome extensions should different way to make windows",
"manifest_version": 3,
"permissions": [
"tabs"
],
"action": {
"default_title": "Chrome Windows",
"default_popup": "index.html"
}
}
Sample Project
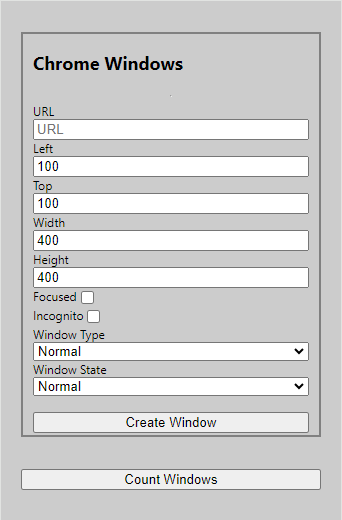
We are going to make an extension that create all types of chrome windows. The user will set the URL, x, y, width, height, incognito, whether its minimized or maximized.
You can find the source to this project here: https://github.com/BuildChromeExtensions/chromewindows
For this extension weโll need 3 files index.html
(popup), manifest.json
and popup.js
(the javascript for the popup).
manifest.json
{
"name": "Chrome Windows",
"version": "1.0.0.0",
"description": "A chrome extensions should different way to make windows",
"manifest_version": 3,
"action": {
"default_title": "Chrome Windows",
"default_popup": "index.html"
}
}
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style type="text/css">
body {
width: 300px;
height: 500px;
background-color: #ccc;
padding: 10px 20px;
display: flex;
flex-direction: column;
justify-content: center;
margin: 0 auto;
}
form {
border: 2px grey solid;
padding: 2px 10px;
display: flex;
flex-direction: column;
justify-content: center;
}
</style>
</head>
<body>
<form>
<h2>Chrome Windows</h2>
<hr />
<!-- URL -->
<label>URL</label>
<input type="url" name="url" placeholder="URL" required />
<!-- Position -->
<label>Left</label>
<input type="number" value="100" min="100" max="2000" name="x" placeholder="Left Position" required />
<label>Top</label>
<input type="number" value="100" min="100" max="2000" name="y" placeholder="Top Position" required />
<!-- Size -->
<label>Width</label>
<input type="number" value="400" min="100" max="2000" name="width" placeholder="Width" required />
<label>Height</label>
<input type="number" value="400" min="100" max="2000" name="height" placeholder="Height" required />
<div style="display: flex;">
<label>Focused</label>
<input type="checkbox" name="focused" />
</div>
<div style="display: flex;">
<label>Incognito</label>
<input type="checkbox" name="incognito" />
</div>
<!-- Window Type -->
<label>Window Type</label>
<select name="windowType" value="normal">
<option value="normal">Normal</option>
<option value="popup">Popup</option>
<!-- <option value="panel">Panel</option> -->
</select>
<!-- Window state -->
<label>Window State</label>
<select name="windowState" value="normal">
<option value="normal">Normal</option>
<option value="minimized">Minimized</option>
<option value="maximized">Maximized</option>
<option value="fullscreen">Fullscreen</option>
</select>
<br />
<button type="submit">Create Window</button>
</form>
<br/><br/>
<button id="window-count">Count Windows</button>
<script type="text/javascript" src="./popup.js">
</script>
</body>
</html>
popup.js
document.getElementById('window-count').onclick = async () => {
console.log('windows')
// https://developer.chrome.com/docs/extensions/reference/api/windows#type-QueryOptions
const query = { windowTypes: ['normal', 'popup'] }
// async/await are supported as well.
// const windows = await chrome.windows.getAll(query)
chrome.windows.getAll(query, (windows) => {
alert(`${windows.length} Windows`);
});
}
document.querySelector('form').onsubmit = (e) => {
e.preventDefault();
const url = e.target.url.value;
//optional fields
const width = parseInt(e.target.width.value);
const height = parseInt(e.target.height.value);
const x = parseInt(e.target.x.value);
const y = parseInt(e.target.y.value);
const windowType = e.target.windowType.value;
const windowState = e.target.windowState.value;
const incognito = e.target.incognito.checked;
const focused = e.target.focused.checked;
//The initial state of the window. The minimized, maximized, and fullscreen states cannot be combined with focused, left, top, width, or height.
if (windowState == 'normal') {
// create window
chrome.windows.create({
url: url,
type: windowType,
width: width,
height: height,
left: x,
top: y,
state: 'normal',
incognito: incognito,
focused: focused
})
} else {
// create window
chrome.windows.create({
url: url,
state: windowState,
incognito: incognito,
})
}
}